How to setup a React PWA project using Vite
— React, Vite, PWA — 6 min read
This tutorial will teach you how to setup a Progressive Web App project with React and Vite.
A Progressive Web App or "PWA" is an application developed using web technologies which can not only be accessed as an online web application but can also be installed as a native app on desktops and phones. It utilizes all the new and enhanced web capabilities to access and use device features on which it is installed and can even work offline. It does all of this while being based off of a single codebase!
Lets dive right in and walk-through the steps required to setup the project.
Step 1: Install Vite+React Starter Project
First lets setup a React starter project using Vite. Enter one of the below commands in your terminal.
npm create vite@latest
#or
yarn create vite
You'll be asked to enter a Project Name, Framework and Variant. You can enter any name for your project. Select "React" as the framework and "JavaScript" as the variant.
You can use the "TypeScript" variant if you want. I haven't personally used it but the steps in this tutorial should work for TypeScript as well. In case you face issues, I have included reference links in each step where you can hopefully get information on how to perform the same operation in TypeScript.
Once finished, in the command prompt, go to the new project directory and then run npm install
followed by npm run dev
. This will start a Vite server on localhost
. If you visit the localhost website, you should have the basic Vite+React app skeleton ready and working.
Reference for more information: How to setup a React Starter Project using Vite
Step 2: Install Vite PWA plugin
Next we'll install the Vite PWA Plugin as a dev dependency.
npm i vite-plugin-pwa -D
Reference: Installing Vite Plugin PWA
Step 3: Install PWA Assets Generator plugin
Next we'll install a plugin called PWA Assets Generator to generate all our PWA assets like the Web Manifest file, Service worker, app icons, splash screen, etc.
npm i @vite-pwa/assets-generator -D
Step 4: Configure the Vite PWA plugin
We need to add some configuration options for the Vite PWA plugin into the vite.config.js
or vite.config.ts
file.
This file will most probably already have a plugins
array property that will have an existing configuration for the React library. So we just need to append the PWA plugin configuration to this array.
import { defineConfig } from 'vite'import react from '@vitejs/plugin-react'import { VitePWA } from 'vite-plugin-pwa';
// https://vitejs.dev/config/export default defineConfig({ plugins: [ react(), VitePWA({ registerType: 'autoUpdate', includeAssets: ['favicon.ico', 'apple-touch-icon.png', 'mask-icon.svg'], manifest: { name: 'Vite PWA Project', short_name: 'Vite PWA Project', theme_color: '#ffffff', icons: [ { src: 'pwa-64x64.png', sizes: '64x64', type: 'image/png' }, { src: 'pwa-192x192.png', sizes: '192x192', type: 'image/png' }, { src: 'pwa-512x512.png', sizes: '512x512', type: 'image/png', purpose: 'any' }, { src: 'maskable-icon-512x512.png', sizes: '512x512', type: 'image/png', purpose: 'maskable' } ], }, }) ],})
Reference:
Step 5: Add an NPM script in package.json
for generating PWA assets
We'll add a script in package.json
that maps to the pwa-assets-generator
command and will kick off the assets generation process. You can append the generate-pwa-assets
property to the existing scripts
property.
{ "scripts": { "generate-pwa-assets": "pwa-assets-generator --preset minimal public/logo.svg" }}
Reference: CLI commands to generate PWA assets.
Step 6: Arrange a logo SVG source file
While it should work for any image type, the PWA plugin documentation strongly suggests using a single SVG file as the source for PWA assets.
So if you already have a logo.svg
file then use that. For the purposes of this tutorial, lets just use the /public/vite.svg
logo file. Duplicate it and name the copy as logo.svg
.
Step 7: Create PWA assets
We're all set! Lets generate PWA assets using the below command.
npm run generate-pwa-assets
When this command completes, you'll notice that the /public
directory will now contain all these new files. These are the PWA assets generated by the plugin.
Step 8: Add <link>
and <meta>
tags for PWA assets in index.html
Next, we need to include these assets into our web page. Append the below tags within <head>
in the index.html
file located in the root of the codebase.
<head> <!-- other tags --> <link rel="icon" href="/favicon.ico" /> <link rel="apple-touch-icon" href="/apple-touch-icon-180x180.png" sizes="180x180" /> <link rel="mask-icon" href="/mask-icon.svg" color="#FFFFFF"> <meta name="theme-color" content="#ffffff"></head>
Reference: CLI commands to generate PWA assets.
Step 9: Build app
Ok pheww...that's it! We're finally ready to build our app and see if it actually works as a PWA.
Build the app with this command.
npm run build
This command will bundle the app and put all the required files into the /dist
directory.
In order to make the server use this directory to serve a localhost website, we'll use Vite's preview feature. Go ahead and run the below command.
npm run preview
Wallah! If you visit the preview localhost URL, you should see the app on your browser's screen and you should also see an install button in your browser's address bar( see the screenshot below ).
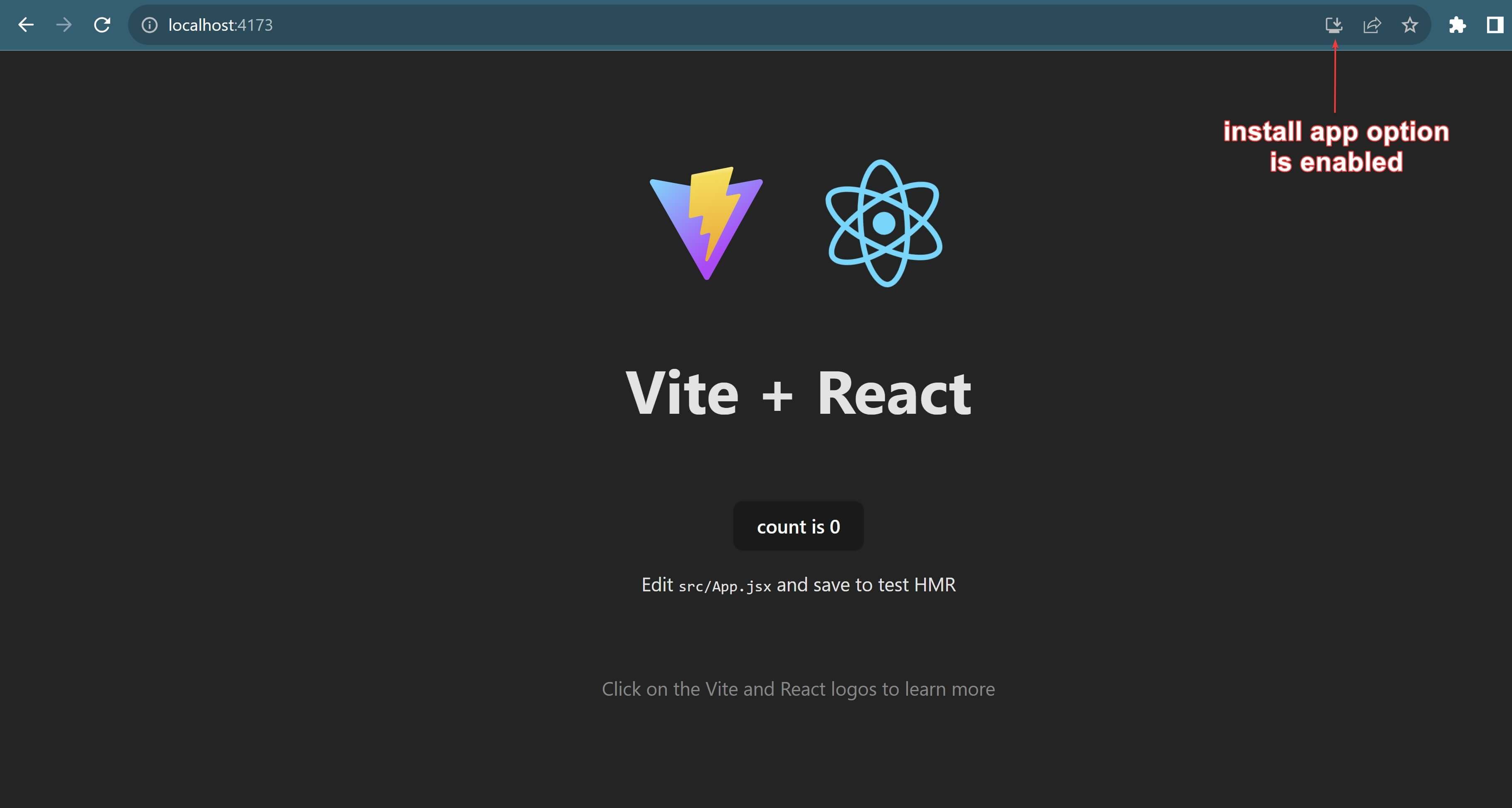
Step 10: Run Lighthouse in Chrome Dev Tools
To make sure our app passes all the criteria for a PWA, we'll run a Lighthouse report in Chrome's Dev tools. See the screenshot below to find out the settings we need to choose to run the report.
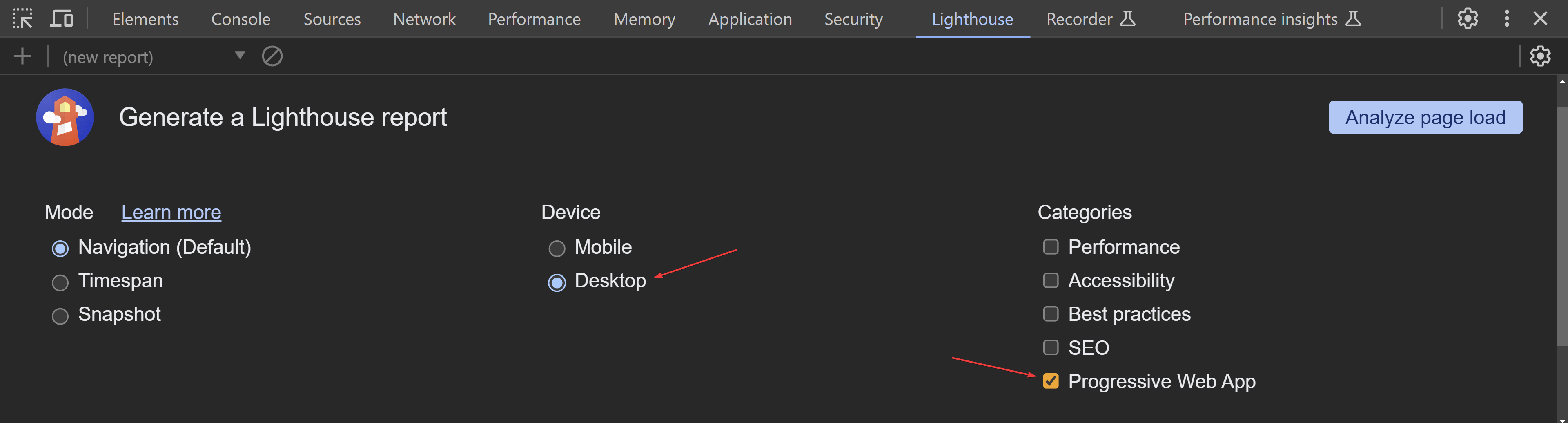
If the criteria is not met, the analysis report will produce errors. If all the criteria are met, the analysis report will show a green check mark like in the screenshot below. This means our app has met all requirements for a PWA and can be installed on various devices.
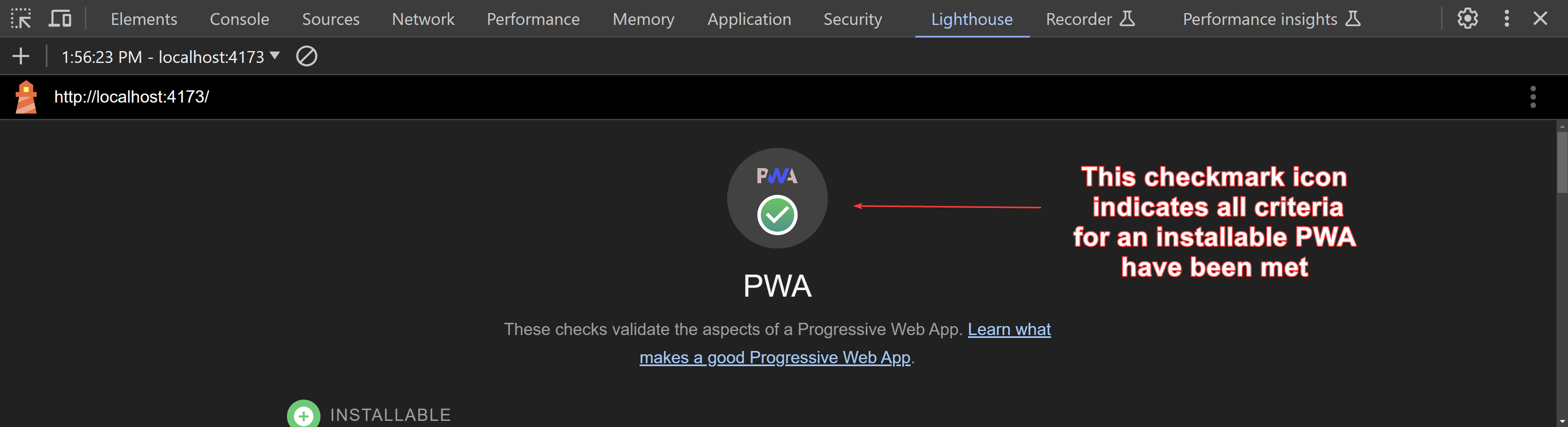
BONUS TIP: Deploying PWA on IIS Server
If you deploy this app on an IIS server, you may face an issue where your .webmanifest
file was not found even though all other files were found. To resolve this issue, make sure your IIS can handle the .webmanifest
extension by adding an entry for .webmanifest
in the global IIS MIME types.
Hope this helps!🙏