How to download files in a NodeJS and ExpressJS REST API | res.sendFile() vs res.download()
— NodeJS, ExpressJS — 2 min read
In this blog post, we'll look at two different ways we can download files from a NodeJS and ExpressJS powered REST API. They are:
- Using
res.sendFile()
- Using
res.download()
Both methods do the same thing i.e. send a file as the API response but there is a bit of difference in how they do it and how clients interpret the responses from both these methods.
If you'd like to be more hands-on while reading this blog post, you can clone or download this github tutorial which has the complete code referenced below.
Using res.sendFile()
For example:
app.get( "/sendfile", ( req, res ) => { res.sendFile( path.join( __dirname + '/images/car.jpg' ) );});
This method will indicate to the client that the file should be opened or displayed rather than downloaded.
If you call this API service from a browser, the browser will directly display the image on the screen.
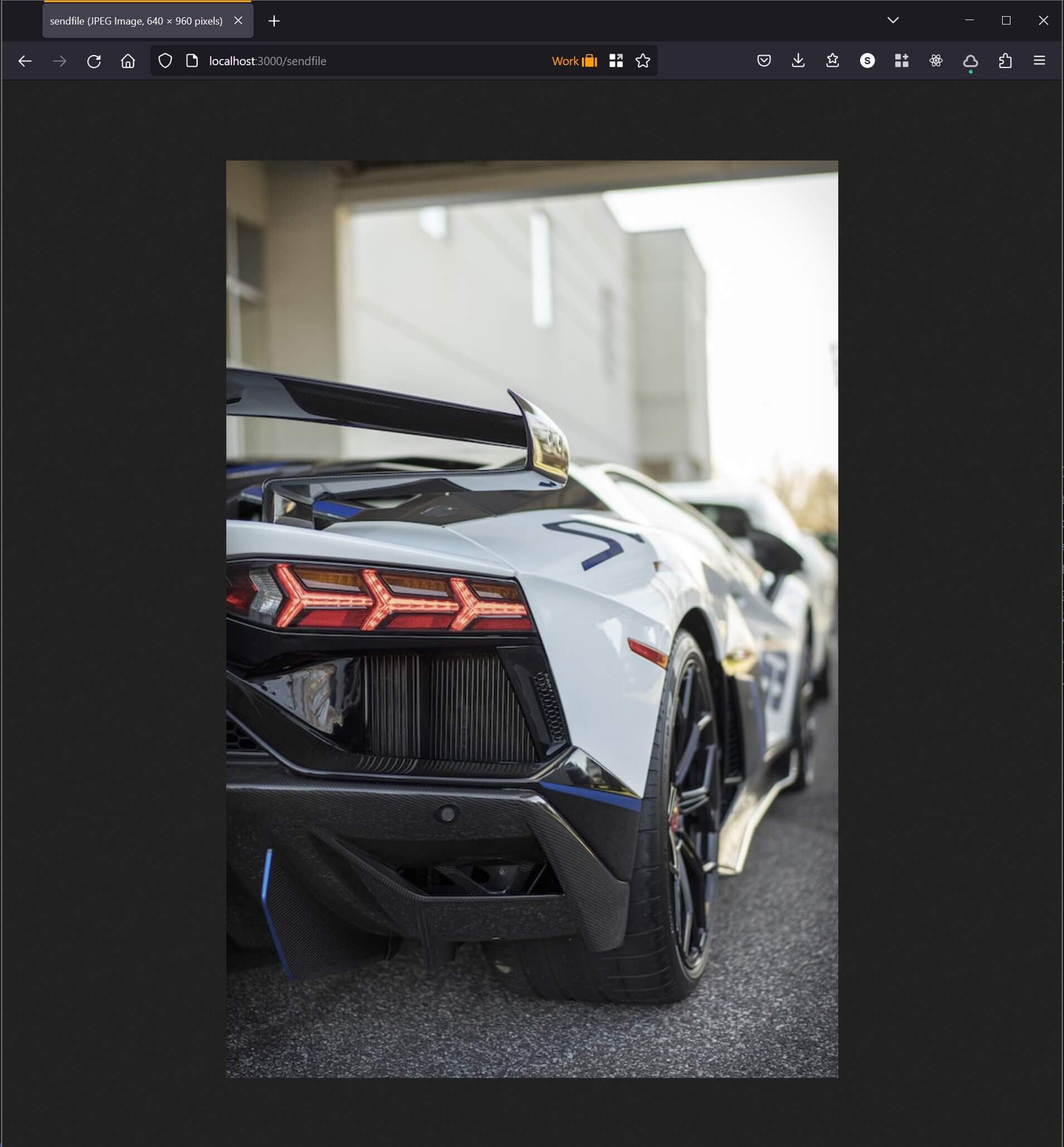
Photo by Brandon Atchison on Unsplash
If you replace the image being sent in the response with a PDF file, the browser will open that PDF file in a new tab.
If you check the headers in the response, you'll see that the Content-Type
header has been automatically set to the mime type of the file sent. In this case, it has been set to image/jpeg
.
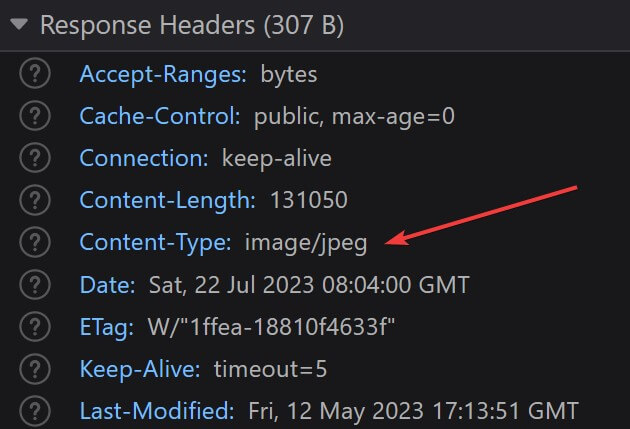
Using res.download()
For example:
app.get( "/download", ( req, res ) => { res.download( path.join( __dirname + '/images/car.jpg' ) );});
This method will indicate to the client that the file should be downloaded and will prompt the user for download.
If you call this API service from a browser, the browser will download this image instead of displaying it on the screen.

If you replace the image being sent in the response with a PDF file, the browser will download the file instead of opening that PDF file in a new tab.
If you check the headers in the response, you'll see that the Content-Type
header has been automatically set to the mime type of the file sent. But additionally, this method also sets the Content-Disposition
header to indicate that this file is an attachment along with the file name.
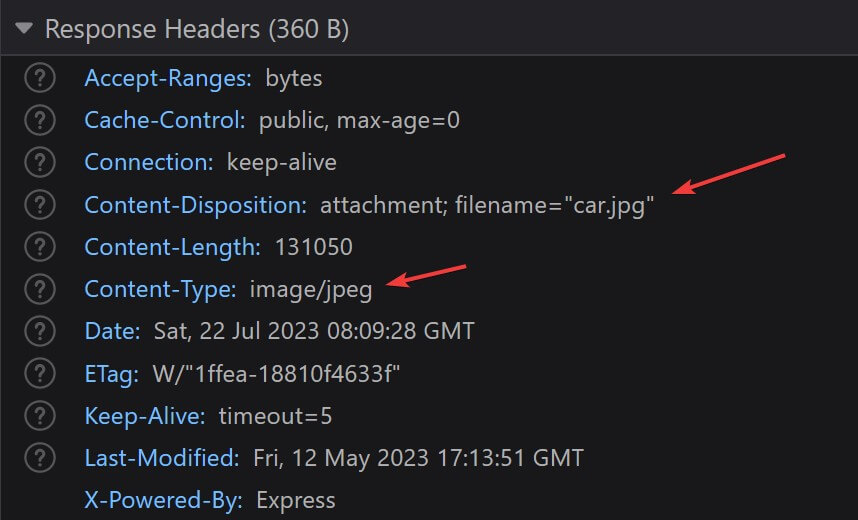
Hope this helps!🙏